Project structure
To explore the structure of your project, start by creating a new project using the following command in the command line:
dotnet new bit-bp --name MyFirstProject
Solution files
The image on the right displays project structure. You will find two solution files in your project: MyFirstProject.sln and MyFirstProject.Web.slnf. The MyFirstProject.Web.slnf file is designed to enhance your development efficiency by providing faster compilation, as it only includes the Backend API and the web version of your application. Conversely, the MyFirstProject.sln file encompasses all projects, such as Android and Windows, and may result in slower opening and build times.Global files
When navigating to the MyFirstProject folder, open the .github directory. Here, you will find the workflows responsible for managing the CI/CD pipeline. The subsequent sections will provide detailed information about these files.The .devcontainer enables seamless integration with GitHub Codespaces when pushing your project to GitHub repository. Demo repository
To enhance the development experience with Visual Studio Code, several configuration files has been included in .vscode directory.
In addition to the .editorconfig and .gitignore files, which have clear purposes, there are two additional files: Clean.bat (Windows) and Clean.sh (macOS/Linux). These scripts are designed to remove all auto-generated files from your project. To understand what are these extra files, please refer to the comments within these scripts. In short, if you face a tough build issue, close your IDE and run the `Clean.bat` or `Clean.sh` file.
There is also a file named global.json, which mitigates potential issues arising from the use of different .NET SDK versions by team members.
Directory.Build.props
In the src folder, which serves as the source of the project, you will find three directories: Client, Server, and Shared, as well as a Directory.Build.props file. Just like csproj files, Directory.Build.props consists of two primary sections: PropertyGroup and ItemGroup.- PropertyGroup: This section specifies properties such as LangVersion. For instance, the following line indicates that the project uses C# 13:
<LangVersion>13.0</LangVersion>
- ItemGroup: This section details items such as NuGet packages used in the project. For example:
<PackageReference Include="Newtonsoft.Json" Version="13.0.3" />
<LangVersion>12.0</langVersion>
This setting enforces the use of C# 12 across all projects. The following sections will provide further details on the Directory.Build.props file and .csproj files.
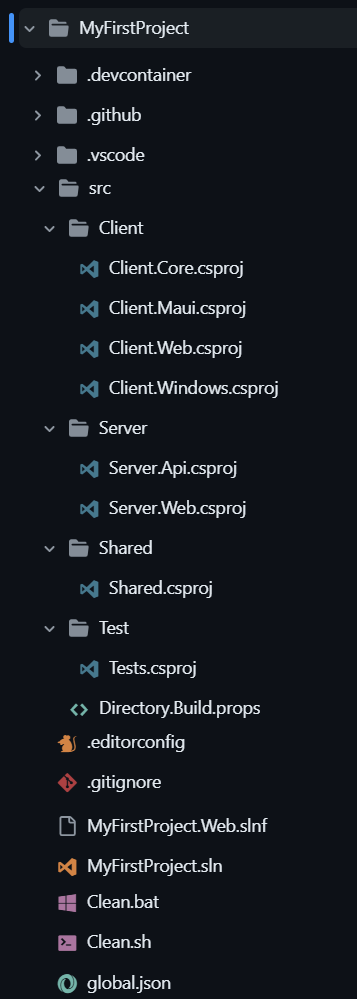
Server Projects
Inside the src/Server folder, there are two projects: Server.Api, Server.Web.- Server.Api: This project is built on Web API, ASP.NET Core Identity, and Entity Framework Core. It includes robust backend features such as social sign-in, two-factor authentication (2FA), Magic Link, and OTP. Despite these advanced features, the architecture remains straightforward, allowing for easy customization similar to a basic ASP.NET Core setup.
- Server.Web: This project references Server.Api by default. Running this project will make the features mentioned in Server.Api section available on port 5000 as well as server components for Blazor pre-rendering and Blazor Server.
Please note that if you choose not to use Blazor Server, Blazor Auto, or Blazor Static SSR in production and instead opt for Blazor WebAssembly without pre-rendering, the Server.Web project remains essential. In our Boilerplate structure, we recommend using Blazor Server mode during development for an enhanced development experience compared to Blazor WebAssembly. When it comes time to publish, you can easily switch to Blazor WebAssembly by making a simple configuration change.
Shared Project
Since both the client and server are written in C#, it is logical to have a shared project that contains code used by both, such as Data Transfer Objects (DTOs) and other common classes.Client Projects
On the client side, the Client.Core project contains various components, including Layouts, Pages, and other essential elements.The Client.Maui project enables the development of applications with 100% percent access to native OS features. Additionally, it supports the use of libraries in Swift, Kotlin, Java, and Objective-C with ease.
In contrast to Client.Maui, which is applicable only to Windows 10 and 11, the Client.Windows project is designed to run on Windows 7, 8, 10, and 11. Additionally, it features an auto-update capability.
Client.Web project can be published as a Blazor WebAssembly Standalone application on platforms such as Cloudflare Pages, Azure Static Web Apps, GitHub Pages, and others, without requiring ASP.NET Core.